Flutter quick start
Target Platforms
This quick start guide shows you how to get Heap setup in a Flutter cross-platform mobile application for iOS and Android.
The SDK is compatible with Flutter 3.16.9 and later.
After completing the setup process, you'll be able to:
- Take full advantage of the Heap API to track custom events and manage user identity.
- Automatically capture a wide variety of user interactions with no additional code required.
Before you begin
The Heap Flutter SDKs have a higher iOS minimum deployment target than the current Flutter Template, iOS 13 instead of iOS 12. To ensure a smooth installation, open ios/Runner.xcworkspace
in Xcode and make sure Minimum Deployments is set to 13.0 or later.
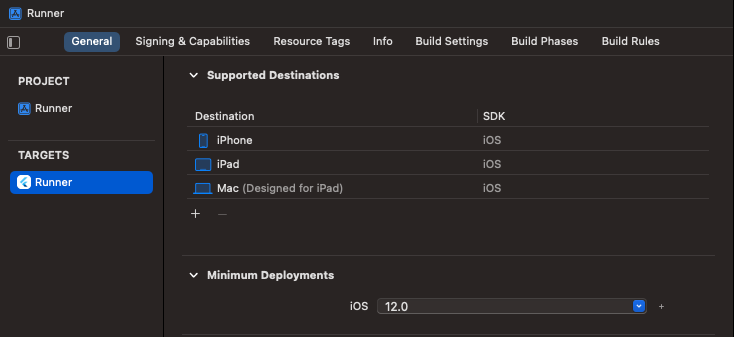
Add Heap dependencies to your project
- Add the Heap dependencies to your
pubspec.yaml
file using theflutter
command:
flutter pub add heap_flutter_bridge &&
flutter pub add heap_flutter_autocapture
- Add the
HeapAutocaptureNavigatorObserver
to all of your widgets that define navigation routes to automatically capture pageviews when navigating in your app.
import 'package:heap_flutter_autocapture/heap_flutter_autocapture.dart';
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
...
navigatorObservers: [
HeapAutocaptureNavigatorObserver(),
],
...
);
}
}
Start the Heap SDK
To enable Heap and start automatically tracking supported UI events, register the autocapture SDK and call startRecording
. We recommend doing this in your main()
method when your application first starts, before your call to runApp()
to ensure all interactions and pageviews are properly captured.
import 'package:heap_flutter_autocapture/heap_flutter_autocapture.dart';
import 'package:heap_flutter_bridge/heap_flutter_bridge.dart';
void main() {
// Must be called before startRecording.
WidgetsFlutterBinding.ensureInitialized();
await initHeap();
runApp(const MyApp());
}
Future<void> initHeap() async {
await HeapAutocapture.register();
await Heap().startRecording('YOUR_APP_ID');
}
Once tracking has started, youāre able to take full advantage of Heapās API to identify users, track custom events, and more. Heap will also automatically start tracking a wide range of user interactions, screen changes, and app version changes without any additional code.
What next?
To find out how to take full advantage of the Heap API to enhance your data capture, check out our mobile tracking guides.
Updated 29 days ago