Flutter Bridge quick start
Target Platforms
This quick start guide shows you how to get Heap set up on an Android or iOS app implemented with Flutter bridge.
Flutter Autocapture
This quick start guide has been deprecated due to the release of the Heap Flutter Autocapture SDK. Please see the updated documentation for Flutter here.
Requirements + Known Limitations
- This SDK requires the following minimum OS versions:
- iOS 12.0
- macOS 10.14
- Android API level 16
- Heap does not currently support Flutter web targets.
Before you begin
HeapFlutterBridge has a higher iOS minimum deployment target than the current Flutter Template, iOS 12 instead of iOS 11. To ensure a smooth installation, open ios/Runner.xcworkspace
in Xcode and make sure Minimum Deployments is set to 12.0 or later.
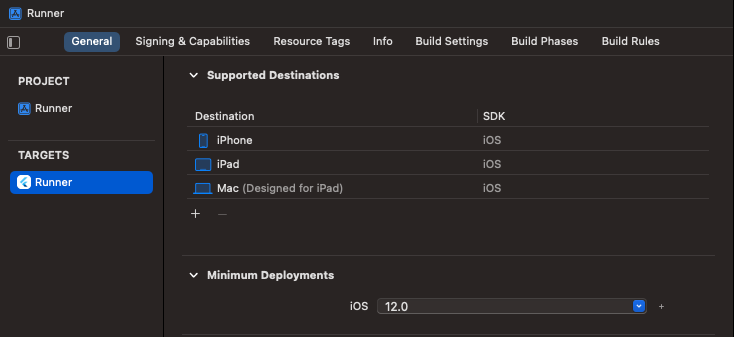
Installation
To install, add heap_flutter_bridge: ^0.1.3
as a dependency in pubspec.yaml
.
...
dependencies:
flutter:
sdk: flutter
heap_flutter_bridge: ^0.1.3
...
After installing, insert the line Heap().startRecording("YOUR_APP_ID")
into your code where you typically insert your startup code, likely in the initState
method of a global state object:
class _MyAppState extends State<MyHomePage> {
@override
void initState() {
Heap().startRecording("YOUR_APP_ID");
super.initState();
}
}
You may also want to increase the log level from info
to debug
to help validate the install:
class _MyAppState extends State<MyHomePage> {
@override
void initState() {
Heap().setLogLevel(HeapLogLevel.debug);
Heap().startRecording("YOUR_APP_ID");
super.initState();
}
}
Log messages will appear in the following places:
- Android:
- Logcat output in Android Studio.
- Debug Console in Visual Studio Code.
- iOS:
- Console debug area in Xcode.
- Console.app when using Visual Studio Code.
- Open the macOS console app.
- Select your simulator or device and start recording.
- Filter logs to the āRunnerā process and the āHeapā category.
- Open the macOS console app.
Tracking events
Tracking can be done with Heap().track(String event, [Map<String, dynamic>? properties])
.
For example Heap().track("incremented_counter");
or Heap().track("incremented_counter", {'value': _counter});
.
All other Heap SDK methods are available on the Heap
object.
Troubleshooting
By default, the Flutter Bridge SDK will automatically target the latest versions of the underlying native SDKs. However, Flutter will attempt to cache the last used version of any native SDKs, so you might need to manually refresh your Android or iOS dependencies to catch the latest version.
Android
To update to the latest version of the underlying native SDK on Android, navigate to your Flutter Android project located in the android
directory and run the following command:
./gradlew clean --refresh-dependencies
iOS and macOS
To update to the latest version of the underlying native SDK on iOS or macOS, navigate to either the Flutter iOS or macOS project in the directory named either ios
or macos
and run the following command:
pod update HeapSwiftCore
Updated 2 months ago